Face Detection
- class tfsdk.TFFacechip
Example of a Facechip:
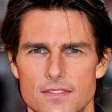
- TFFacechip.save_image(self: tfsdk.TFFacechip, filepath: str) None
Save the face chip to disk.
- Parameters:
filepath - The filepath where the face chip should be saved, including the image extension.
- TFFacechip.load_image(self: tfsdk.TFFacechip, filepath: str, gpu_memory: bool = False, gpu_index: int = 0) tfsdk.ERRORCODE
Load the face chip from disk.
- Parameters:
filepath - The filepath of the facechip to load.
gpu_memory - Read the image into GPU memory. This should be set to true when running GPU inference.
gpu_index - The GPU index.
- TFFacechip.get_height(self: tfsdk.TFFacechip) int
Get the face chip height in pixels.
- Returns:
int - The face chip height in pixels.
- TFFacechip.get_width(self: tfsdk.TFFacechip) int
Get the face chip width in pixels.
- Returns:
int - The face chip width in pixels.
- TFFacechip.as_numpy_array(self: tfsdk.TFFacechip) numpy.ndarray[numpy.uint8]
Get the facechip as a numpy array.
- Returns:
numpy.ndarray[numpy.uint8] - The facechip as a numpy array in BGR format.
- SDK.detect_faces(self: tfsdk.SDK, tf_image: tfsdk.TFImage) Tuple[tfsdk.ERRORCODE, List[tfsdk.FaceBoxAndLandmarks]]
Detect all the faces in the image and return the bounding boxes and facial landmarks. Use the
FACEDETECTIONFILTER
configuration option to filter the detected faces. Refer to our FAQ page to understand the impact of face height on similarity score. The face detector is able to detect faces in the following dynamic height range. Smallest detectable face = ((the larger of your image dimensions) / 640 * 20) pixels. Largest detectable face = (your image height) pixels.- Parameters:
tf_image - The input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.- Returns:
tfsdk.ERRORCODE
- The error code.List[
tfsdk.FaceBoxAndLandmarks
] - The detected faces. If no faces are found, the list will be empty. The detected faces are sorted in order of descending face score.
- SDK.detect_largest_face(self: tfsdk.SDK, tf_image: tfsdk.TFImage) Tuple[tfsdk.ERRORCODE, bool, tfsdk.FaceBoxAndLandmarks]
Detect the largest face in the image by area. Use the
FACEDETECTIONFILTER
configuration option to filter the detected faces. Refer to our FAQ page to understand the impact of face height on similarity score. The face detector is able to detect faces in the following dynamic height range. Smallest detectable face = ((the larger of your image dimensions) / 640 * 20) pixels. Largest detectable face = (your image height) pixels.- Parameters:
tf_image - The input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.- Returns:
tfsdk.ERRORCODE
- The error code.bool - Indicates if a face was detected.
tfsdk.FaceBoxAndLandmarks
- The detected face.
- SDK.get_face_landmarks(*args, **kwargs)
Overloaded function.
get_face_landmarks(self: tfsdk.SDK, tf_images: List[tfsdk.TFImage], face_box_and_landmarks_vec: List[tfsdk.FaceBoxAndLandmarks]) -> Tuple[tfsdk.ERRORCODE, List[Annotated[List[tfsdk.Point], FixedSize(106)]]]
Obtain the 106 face landmarks. This batch processing method increases throughput when using GPU inference.
- Parameters:
tf_images - Array of input
tfsdk.TFImage
.face_box_and_landmarks_vec - Array of
tfsdk.FaceBoxAndLandmarks
.
- Returns:
tfsdk.ERRORCODE
- The error code.List[Annotated[List[tfsdk.Point], FixedSize(106)]] - Array of lists of the 106 face landmark points.
get_face_landmarks(self: tfsdk.SDK, tf_image: tfsdk.TFImage, face_box_and_landmarks: tfsdk.FaceBoxAndLandmarks) -> Tuple[tfsdk.ERRORCODE, Annotated[List[tfsdk.Point], FixedSize(106)]]
Obtain the 106 face landmarks.
- Parameters:
tf_image - The input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.face_box_and_landmarks -
tfsdk.FaceBoxAndLandmarks
returned bytfsdk.SDK.detect_faces()
ortfsdk.SDK.detect_largest_face()
.
- Returns:
tfsdk.ERRORCODE
- The error code.Annotated[List[tfsdk.Point], FixedSize(106)] - List of the 106 face landmark points.
The order of the face landmarks:
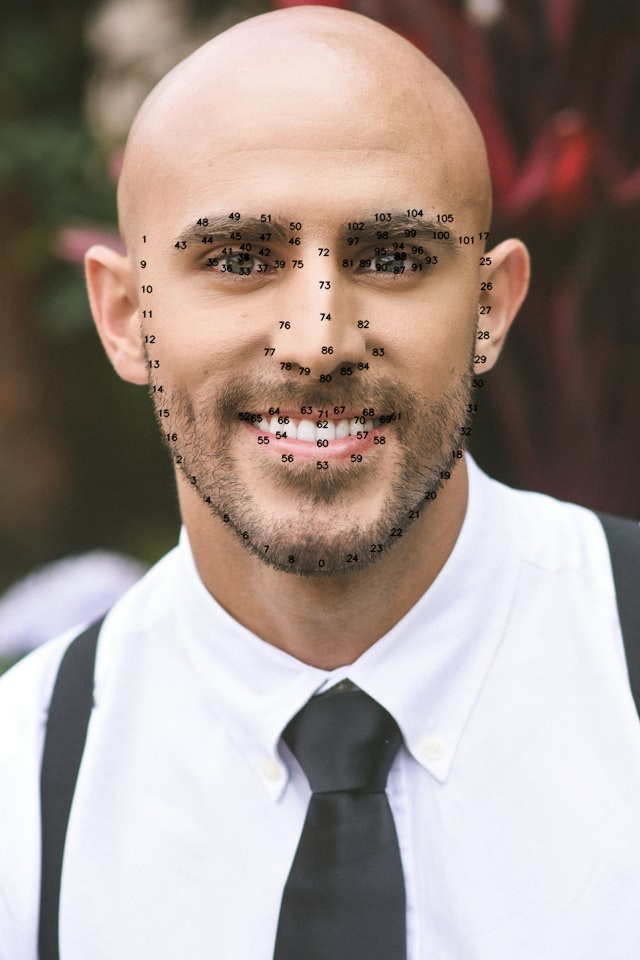
- SDK.extract_aligned_face(self: tfsdk.SDK, tf_image: tfsdk.TFImage, face_box_and_landmarks: tfsdk.FaceBoxAndLandmarks, margin_left: int = 0, margin_top: int = 0, margin_right: int = 0, margin_bottom: int = 0, scale: float = 1.0) Tuple[tfsdk.ERRORCODE, tfsdk.TFFacechip]
Extract the aligned face chip. Changing the margins and scale will change the face chip size. If using the face chip with Trueface algorithms (ex face recognition), do not change the default margin and scale values.
- Parameters:
tf_image - The input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.face_box_and_landmarks - The
tfsdk.FaceBoxAndLandmarks
returned bytfsdk.SDK.detect_largest_face()
ortfsdk.SDK.detect_faces()
.margin_left - Adds a margin to the left side of the face chip (default = 0).
margin_top - Adds a margin to the top side of the face chip (default = 0).
margin_right - Adds a margin to the right side of the face chip (default = 0).
margin_bottom - Adds a margin to the bottom side of the face chip (default = 0).
scale - Changes the scale of the face chip (default = 1).
- Returns:
tfsdk.ERRORCODE
- The error code.tfsdk.TFFacechip
- The aligned face chip.
- SDK.estimate_head_orientation(self: tfsdk.SDK, tf_image: tfsdk.TFImage, face_box_and_landmarks: tfsdk.FaceBoxAndLandmarks, landmarks: Annotated[List[tfsdk.Point], FixedSize(106)]) Tuple[tfsdk.ERRORCODE, tfsdk.HeadOrientation]
Estimate the head orientation. Refer to our FAQ page to understand the impact of head orientation on similarity score.
- Parameters:
tf_image - The input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.face_box_and_landmarks - The
tfsdk.FaceBoxAndLandmarks
.landmarks - The detailed landmarks returned by
tfsdk.SDK.get_face_landmarks()
.
- Returns:
tfsdk.ERRORCODE
- The error code.tfsdk.HeadOrientation
- The head orientation.
- class tfsdk.Point
- to_dict(self: tfsdk.Point) dict
Return a dictionary representation of the object.
- property x
Coordinate along the horizontal axis, or pixel column.
- property y
Coordinate along the vertical axis, or pixel row.
- class tfsdk.FaceBoxAndLandmarks
-
- get_area(self: tfsdk.FaceBoxAndLandmarks) float
Get the area of the face box in pixels squared.
- get_height(self: tfsdk.FaceBoxAndLandmarks) float
Get the the height of the face box in pixels.
- property landmarks
The list of facial landmark points (
Point
) in this order: subject right eye, subject left eye, nose, subject right mouth corner, subject left mouth corner.
- property score
Likelihood of this being a true positive.
- to_dict(self: tfsdk.FaceBoxAndLandmarks) dict
Return a dictionary representation of the object.
The order of the face landmarks:
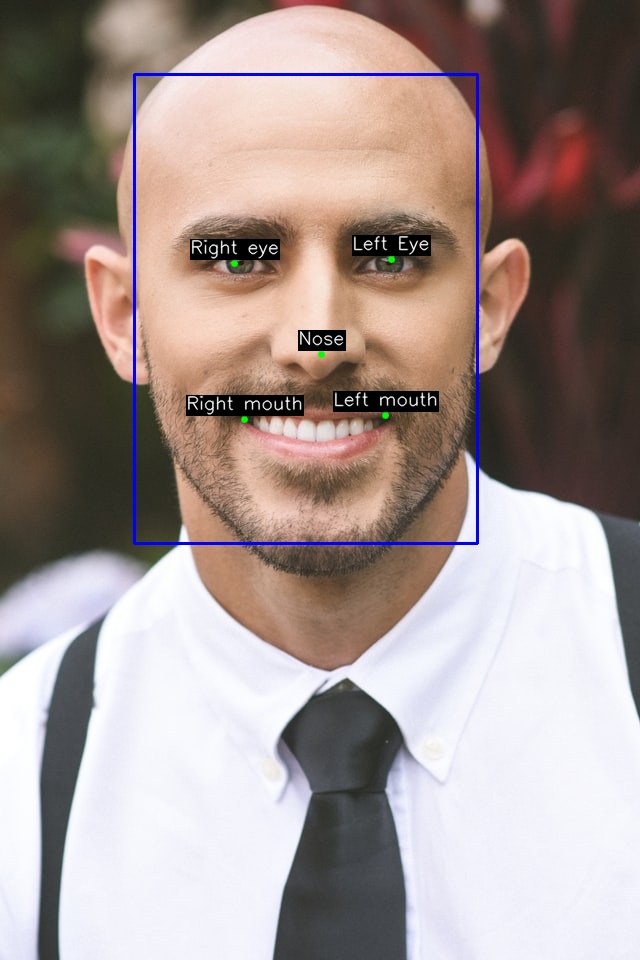
- class tfsdk.HeadOrientation
- property pitch
The rotation angle around the image’s transverse axis, in radians.
- property roll
The rotation angle around the image’s longitudinal axis, in radians.
- property rotation_vec
The rotation vector, can be passed to
tfsdk.SDK.draw_head_orientation_box()
method.
- property translation_vec
The translation vector, can be passed to
tfsdk.SDK.draw_head_orientation_box()
method.
- property yaw
The rotation angle around the image’s vertical axis, in radians.