Face Detection¶
-
class Facechip¶
Class which is used to manage a face chip image. A face chip represents and aligned and cropped face image. A Facechip can be in CPU memory or GPU memory.
Example of a Facechip:
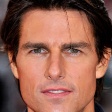
-
void Trueface::Facechip::saveImage(const std::string &filepath)¶
Save the face chip to disk.
- Parameters
filepath – the filepath where the face chip should be saved, including the image extension.
-
ErrorCode Trueface::Facechip::loadImage(const std::string &filepath, bool gpuMemory = false, int gpuIndex = 0)¶
Load the face chip from disk.
- Parameters
filepath – the filepath of the facechip to load.
gpuMemory – read the image into GPU memory. This should be set to true when running GPU inference.
gpuIndex – the GPU index.
-
unsigned int Trueface::Facechip::getHeight() const¶
Get the face chip height in pixels.
- Returns
Returns the face chip height in pixels.
-
unsigned int Trueface::Facechip::getWidth() const¶
Get the face chip width in pixels.
- Returns
Returns the face chip width in pixels.
-
unsigned int Trueface::Facechip::getStride() const¶
Get the GPU stride for GPU images.
- Returns
Returns the GPU stride for the Facechip.
-
bool Trueface::Facechip::isGPUImage() const¶
Is the face chip in GPU memory.
- Returns
Returns true if the face chip is in GPU memory.
-
uint8_t *Trueface::Facechip::getData() const¶
Get the decoded image buffer.
- Returns
Returns the decoded image buffer.
-
ErrorCode Trueface::SDK::detectFaces(const TFImage &tfImage, std::vector<FaceBoxAndLandmarks> &faceBoxAndLandmarks)¶
Detect all the faces in the image. Use the ConfigurationOptions::fdFilter to filter the detected faces.
Refer to our FAQ page to understand the impact of face height on similarity score.
The face detector is able to detect faces in the following dynamic height range. Smallest detectable face = ((the larger of your image dimensions) / 640 * 20) pixels. Largest detectable face = (your image height) pixels.
- Parameters
tfImage – [in] the input image returned by preprocessImage().
faceBoxAndLandmarks – [out] a vector of FaceBoxAndLandmarks representing each of the detected faces. If not faces are found, the vector will be empty. The detected faces are sorted in order of descending face score.
- Returns
error code, see ErrorCode.
-
ErrorCode Trueface::SDK::detectLargestFace(const TFImage &tfImage, FaceBoxAndLandmarks &faceBoxAndLandmarks, bool &found)¶
Detect the largest face in the image by area. Use the ConfigurationOptions::fdFilter to filter the detected faces.
Refer to our FAQ page to understand the impact of face height on similarity score.
The face detector is able to detect faces in the following dynamic height range. Smallest detectable face = ((the larger of your image dimensions) / 640 * 20) pixels. Largest detectable face = (your image height) pixels.
- Parameters
tfImage – [in] the input image returned by preprocessImage().
faceBoxAndLandmarks – [out] the FaceBoxAndLandmarks containing the landmarks and bounding box of the largest detected face in the image.
found – [out] whether a face was found in the image.
- Returns
error code, see ErrorCode.
-
ErrorCode Trueface::SDK::getFaceLandmarks(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, Landmarks &landmarks)¶
Obtain the 106 face landmarks.
- Parameters
tfImage – [in] the input image returned by preprocessImage().
faceBoxAndLandmarks – [in] FaceBoxAndLandmarks returned by detectFaces() or detectLargestFace().
landmarks – [out] an array of 106 face landmark points.
- Returns
error code, see ErrorCode.
The order of the face landmarks:
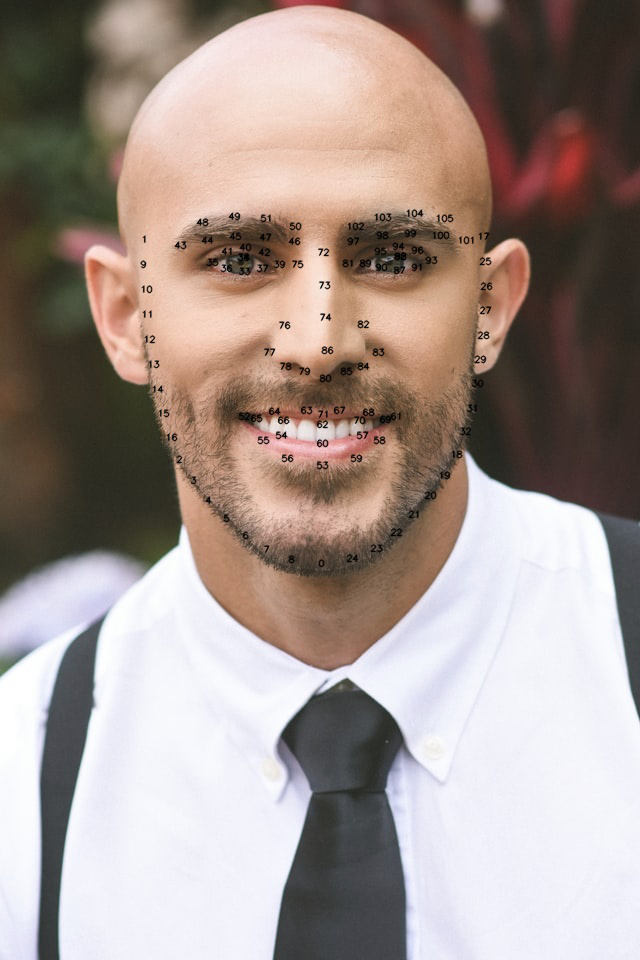
-
ErrorCode Trueface::SDK::extractAlignedFace(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, TFFacechip &tfFacechip, int marginLeft = 0, int marginTop = 0, int marginRight = 0, int marginBottom = 0, float scale = 1.0)¶
Align the the detected face to be optimized for passing to feature extraction. If using the face chip with Trueface algorithms (ex face recognition), do not change the default margin and scale values.
- Parameters
tfImage – [in] the input image returned by preprocessImage().
faceBoxAndLandmarks – [in] the FaceBoxAndLandmarks returned by detectLargestFace() or detectFaces().
tfFacechip – [out] A TFFacechip to store the output facechip.
marginLeft – [in] adds a margin to the left side of the face chip.
marginTop – [in] adds a margin to the top side of the face chip.
marginRight – [in] adds a margin to the right side of the face chip.
marginBottom – [in] adds a margin to the bottom side of the face chip.
scale – [in] changes the scale of the face chip.
- Returns
error code, see ErrorCode.
-
ErrorCode Trueface::SDK::estimateHeadOrientation(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, const Landmarks &landmarks, float &yaw, float &pitch, float &roll, std::array<double, 3> &rotationVec, std::array<double, 3> &translationVec)¶
Estimate the head orientation. Refer to our FAQ page to understand the impact of face orientation on similarity score.
- Parameters
tfImage – [in] the input image returned by preprocessImage().
faceBoxAndLandmarks – [in] the face box and landmarks, returned by detectLargestFace().
landmarks – [in] the detailed face landmarks, returned by getFaceLandmarks().
yaw – [out] the rotation angle around the image’s vertical axis, in radians.
pitch – [out] the rotation angle around the image’s transverse axis, in radians.
roll – [out] the rotation angle around the image’s longitudinal axis, in radians.
rotationVec – [out] the rotation vector, can be passed to drawHeadOrientationBox() method.
translationVec – [out] the rotation translation vector, can be passed to drawHeadOrientationBox() method.
- Returns
error code, see ErrorCode.
-
template<typename T>
struct Trueface::Point¶
-
struct Trueface::FaceBoxAndLandmarks¶
Public Functions
-
float getHeight() const¶
Returns the height of the face box in pixels.
-
float getArea() const¶
Returns the area of the face box in pixels squared.
-
float getHeight() const¶
The order of the face landmarks:
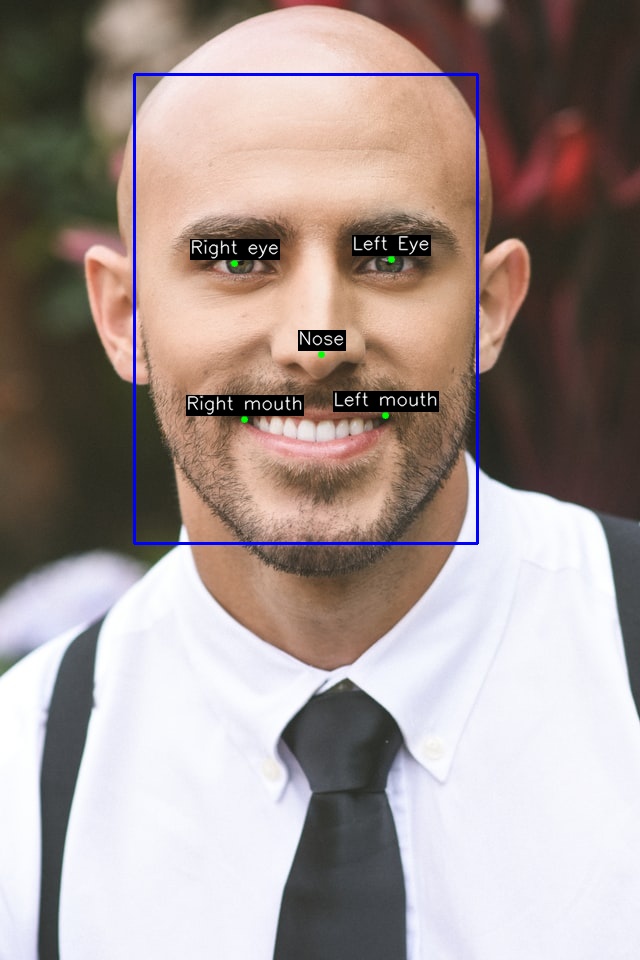