Spoof Detection
Active Spoof
Active spoof works by observing the geometry and perspective of a face as it moves closer to a camera. Active spoof requires two input images, one far and one close, and is an involved process. Therefore, please be sure to consult the sample code for a demonstration of proper usage.
-
ErrorCode Trueface::SDK::checkSpoofImageFaceSize(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, ActiveSpoofStage activeSpoofStage)
Ensures that the face size meets the requirements for active spoof. Must check return value of function! Active spoof works by analyzing the way a persons face changes as they move closer to a camera. The active spoof solution therefore expects the face a certain distance from the camera. In the far image, the face should be about 18 inches from the camera, while in the near image, the face should be 7-8 inches from the camera. This function must be called before calling detectActiveSpoof().
- Parameters:
tfImage – [in] The input image returned by preprocessImage().
faceBoxAndLandmarks – [in] The face on which to run active spoof detection.
activeSpoofStage – [in] The stage of the image, either near stage or far stage.
- Returns:
Error code, see ErrorCode. If
ErrorCode::NO_ERROR
is returned, then the image is eligible for active spoof detection. IfErrorCode::FACE_TOO_CLOSE
orErrorCode::FACE_TOO_FAR
is returned, the image is not eligible for active spoof detection.
-
ErrorCode Trueface::SDK::detectActiveSpoof(const Landmarks &nearFaceLandmarks, const Landmarks &farFaceLandmarks, float &spoofScore, SpoofLabel &spoofPrediction)
Detect if there is a presentation attack attempt. Must call checkSpoofImageFaceSize() on both input faces before calling this function.
- Parameters:
nearFaceLandmarks – [in] The face landmarks of the near face, obtained by calling getFaceLandmarks().
farFaceLandmarks – [in] The face landmarks of the far face, obtained by calling getFaceLandmarks().
spoofScore – [out] The output spoof score. If the spoof score is above the threshold, then it is classified as a real face. If the spoof score is below the threshold, then it is classified as a fake face.
spoofPrediction – [out] The predicted spoof result, using a spoofScore threshold of 1.05.
- Returns:
Error code, see ErrorCode.
Passive Spoof
Passive spoof is meant to be used for selfie-style images from smartphones or from laptop webcams, and should not be used with CCTV cameras / cameras at a distance. Unlike active spoof which requires two input images (one close and one far), passive spoof requires only a single input image. This being said, there are still strict requirements for said input image:
Face must be at least 100 pixels in height. Face height must also be between 0.323 and 0.55 of the total image height.
The face must be centered vertically and horizontally within the frame for portrait images. Landscape images are more permissive for horizontal centering as a portrait region is cropped around the face.
The face must have neutral yaw, pitch, and roll, and must be facing the camera directly.
The eyes must be open.
User must not be wearing a mask.
The image must not be overly bright or dark.
If any of the above criteria are not met, the method will return an error code. It is therefore imperative to check the return value of the function. Please consult the sample code for proper usage of this method. We advise running this method on the front end so that immediate feedback can be provided to the user until they submit a conforming image. We also advise running multiple images through the spoof detector (4-6) and then taking the average result.
-
ErrorCode Trueface::SDK::detectSpoof(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, SpoofLabel &result, float &spoofScore, float threshold = 0.75)
Detect if there is a presentation attack attempt. Passive spoof detection only works with “selfie-style”, meaning the face must be up close and take up most of the frame.
- Parameters:
tfImage – [in] The input image returned by preprocessImage().
faceBoxAndLandmarks – [in] FaceBoxAndLandmarks returned by detectFaces() or detectLargestFace().
result – [out] The SpoofLabel results for the face image.
spoofScore – [out] The probability that the image is a spoof attempt (1 indicates real image, 0 indicates spoof attempt).
threshold – [in] The spoof score threshold above which it is considered a real image.
- Returns:
Error code, see ErrorCode.
-
ErrorCode Trueface::SDK::detectSpoof(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, const HeadOrientation &headOrientation, const BlinkState &blinkState, const MaskLabel &maskLabel, SpoofLabel &result, float &spoofScore, float threshold = 0.75)
Detect if there is a presentation attack attempt. Passive spoof detection only works with “selfie-style”, meaning the face must be up close and take up most of the frame.
- Parameters:
tfImage – [in] The input image returned by preprocessImage().
faceBoxAndLandmarks – [in] FaceBoxAndLandmarks returned by detectFaces() or detectLargestFace().
headOrientation – [in] The head orientation, returned by estimateHeadOrientation().
blinkState – [in] The output of the blink prediction, as returned by detectBlink()
maskLabel – [in] The predicted MaskLabel for face image, as returned by detectMask()
result – [out] The SpoofLabel results for the face image.
spoofScore – [out] The probability that the image is a spoof attempt (1 indicates real image, 0 indicates spoof attempt).
threshold – [in] The spoof score threshold above which it is considered a real
- Returns:
Error code, see ErrorCode.
Below are two examples of conforming images, one landscape, and one portrait:
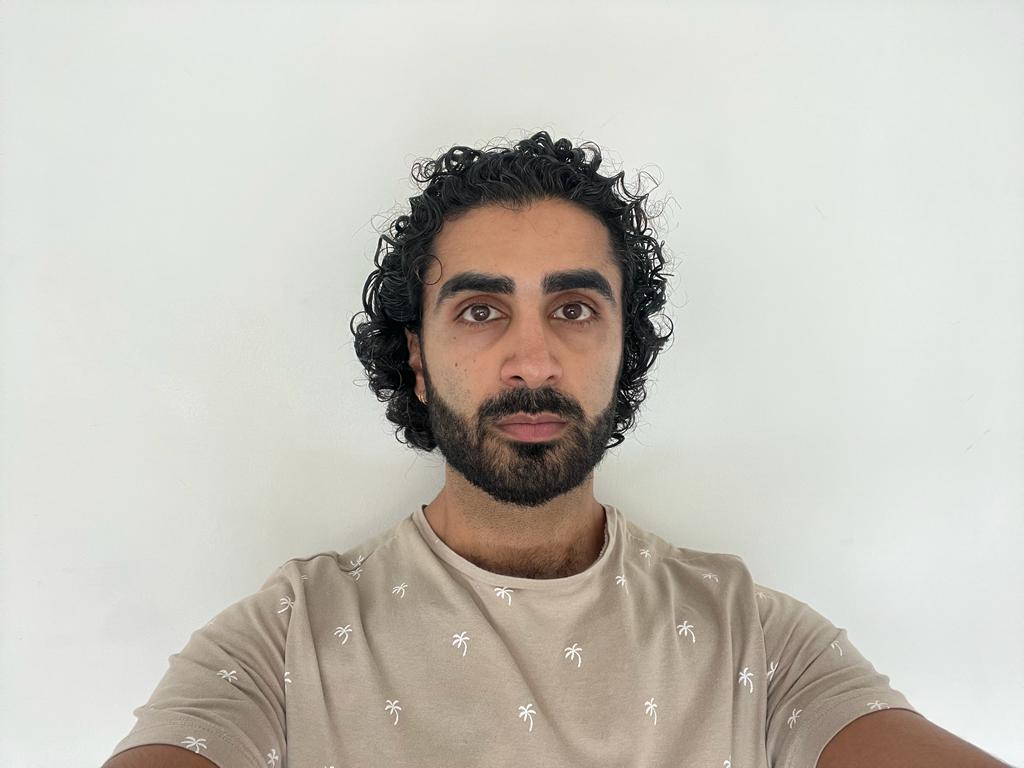
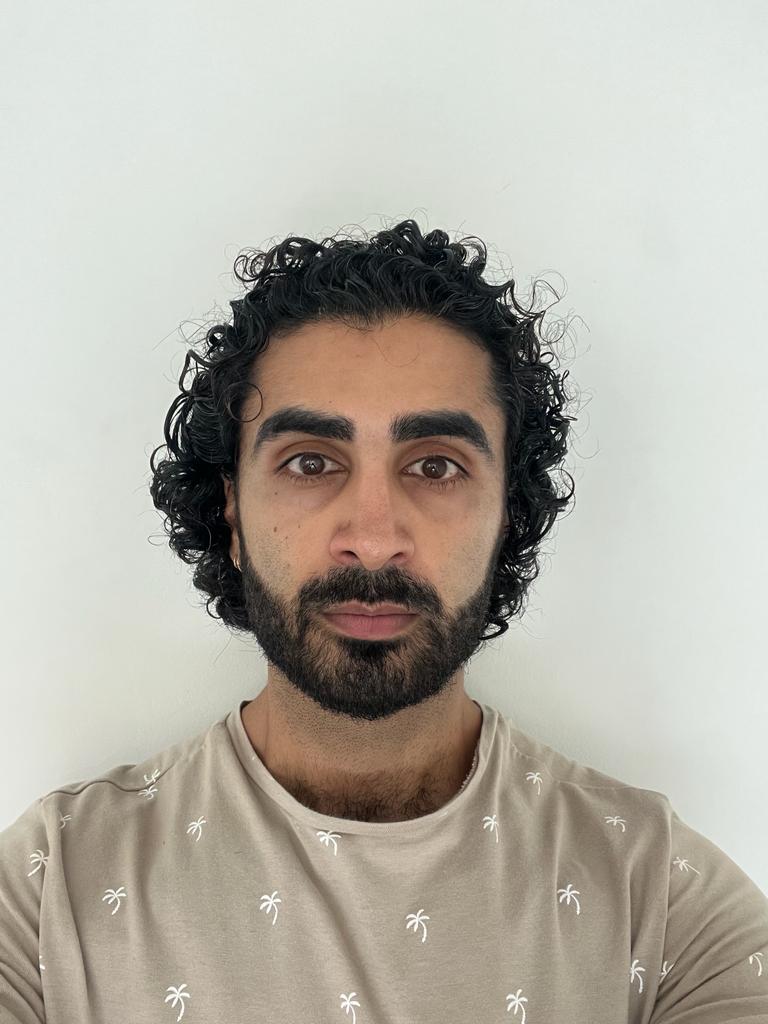
Below are four examples of non-conforming images:
Face too far:
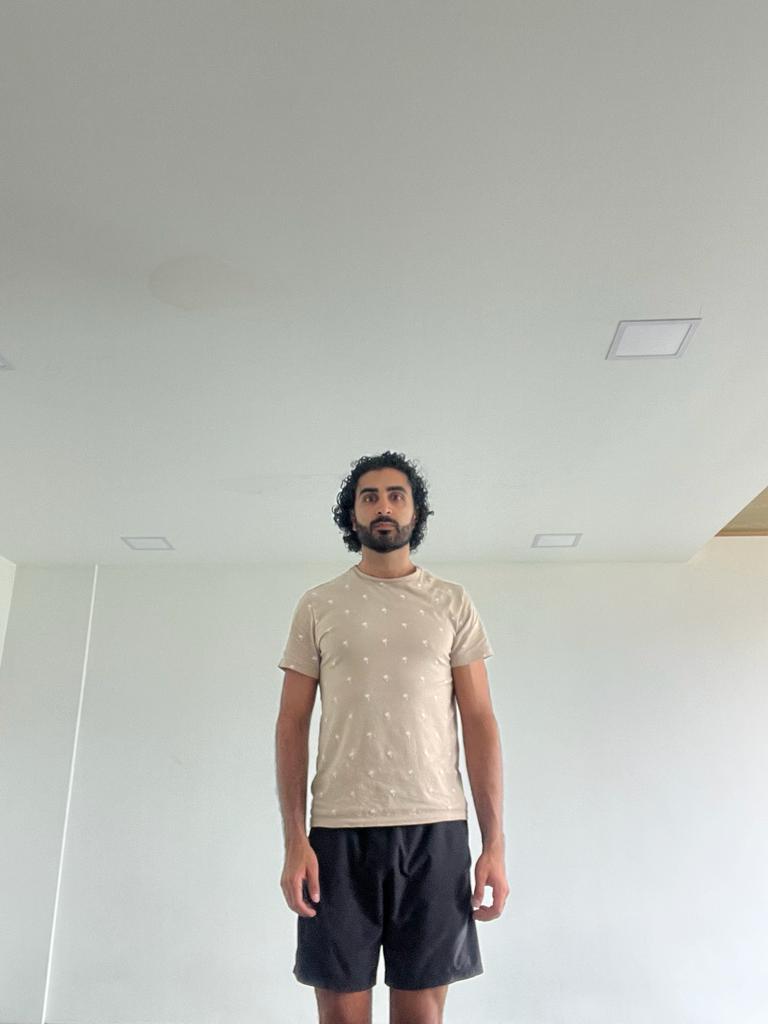
Face too close:
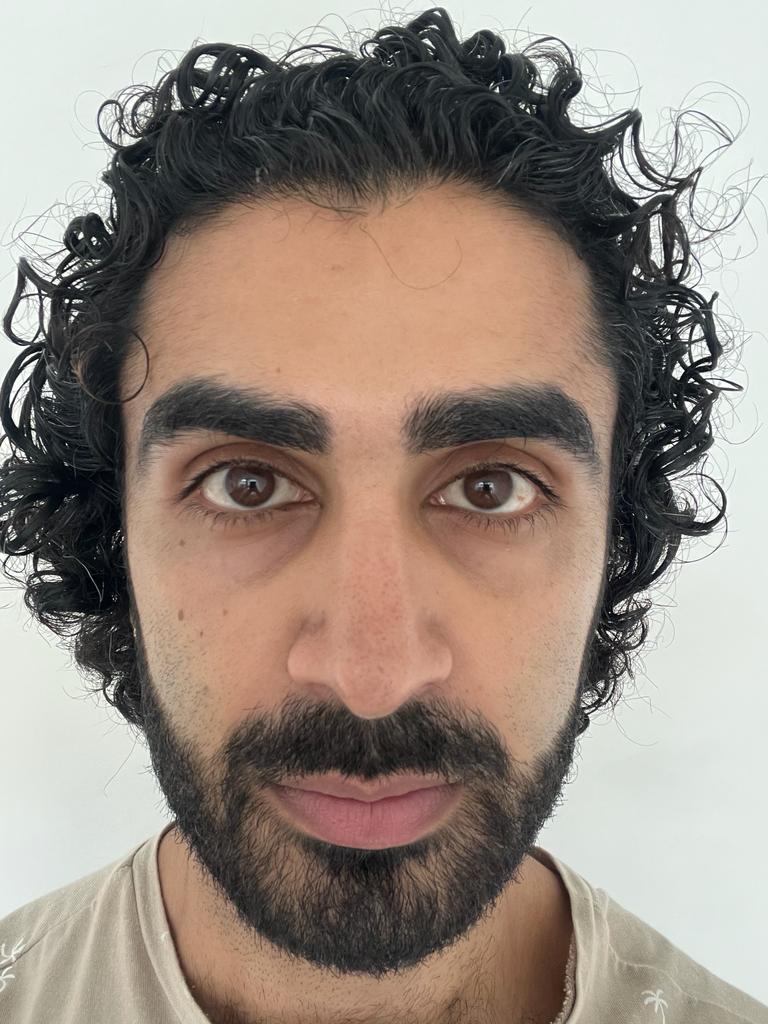
Face yaw angle too extreme:
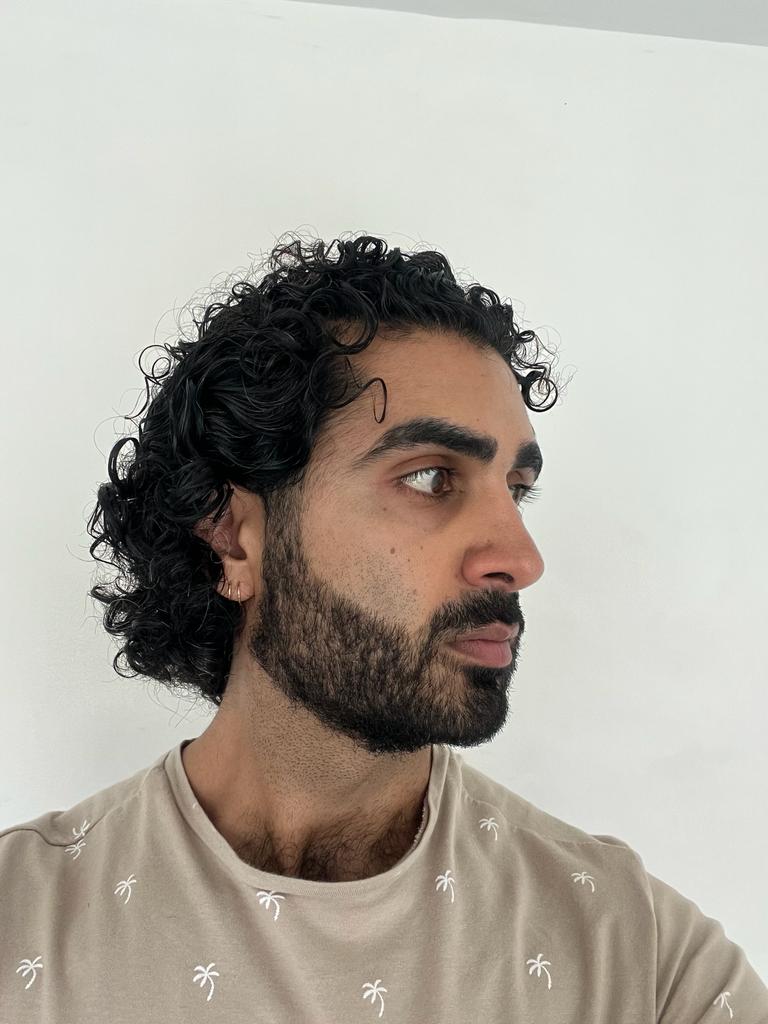
Face roll angle too extreme:
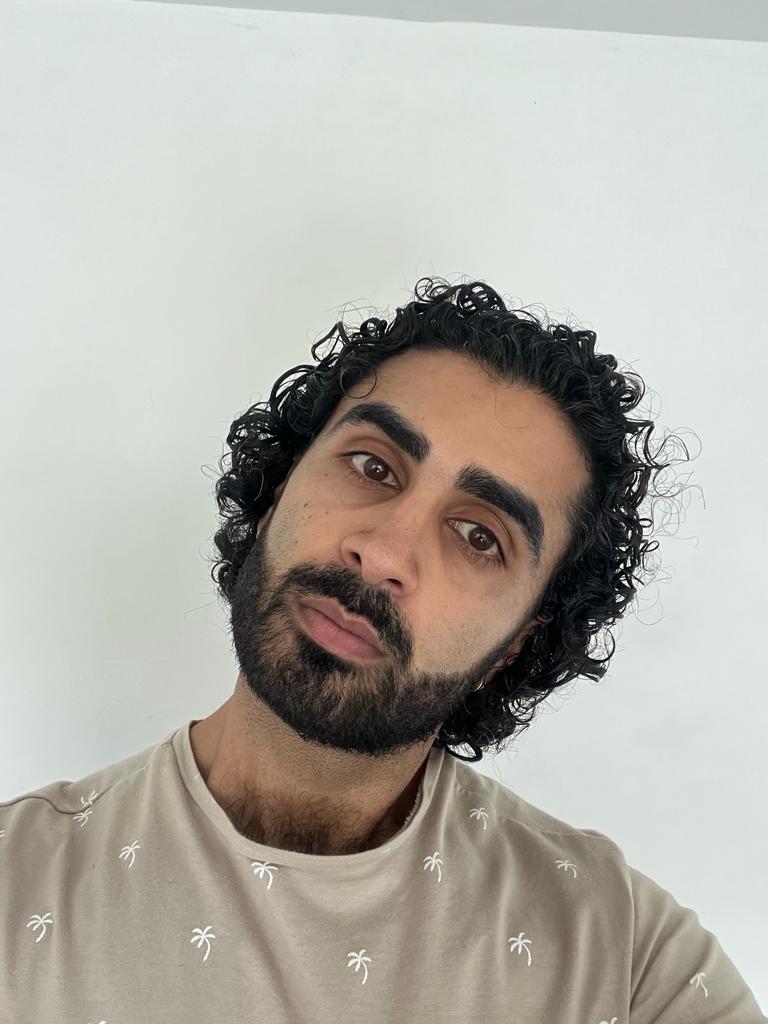
Image too bright:
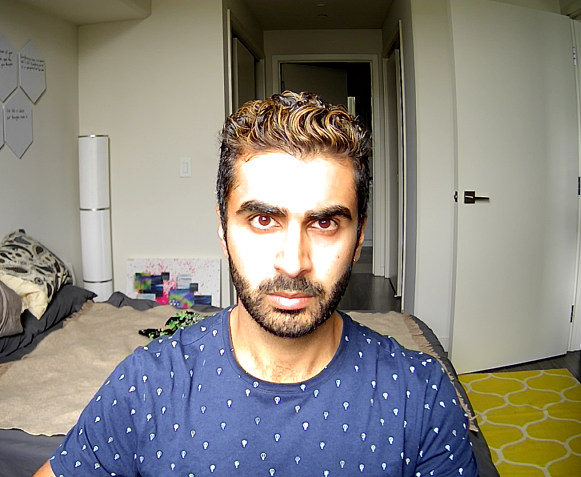