Image Annotation¶
Methods for annotating images with face landmarks, bounding boxes, face recognition labels, and more. After annotating an image, you must not run inference functions on that image. You can call multiple annotation methods on the same image.
- SDK.draw_face_box_and_landmarks(self: tfsdk.SDK, tf_image: tfsdk.TFImage, face_box_and_landmarks: tfsdk.FaceBoxAndLandmarks, include_landmarks: bool = False, color: tfsdk.ColorRGB = <tfsdk.ColorRGB object at 0x7f0375383f70>, scale: int = 2) → tfsdk.ERRORCODE¶
Draw face detection bounding box and 5 keypoint landmarks. Note, you should not run any inference functions on the image after calling this method.
- Parameters
- Parameters
tf_image – the input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.face_box_and_landmarks – the face to annotate.
include_landmarks – indicates if the 5 keypoint landmarks should also be drawn.
color – the color to use for the annotation (default = ColorRGB(0, 255, 255).
- Scale
scale factor for the bounding box thickness. Increase scale factor for higher resolution images.
- Returns
Error code, see
ERRORCODE
.
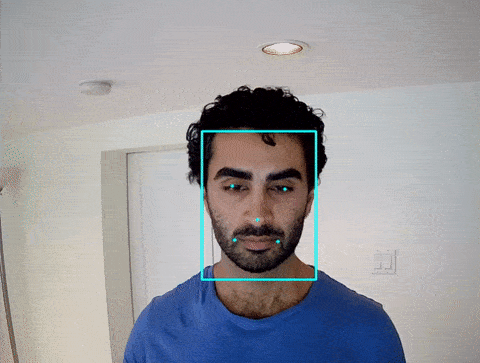
- SDK.draw_face_landmarks(self: tfsdk.SDK, tf_image: tfsdk.TFImage, landmarks: Annotated[List[tfsdk.Point], FixedSize(106)], color: tfsdk.ColorRGB = <tfsdk.ColorRGB object at 0x7f037538e130>, scale: int = 2) → tfsdk.ERRORCODE¶
Draw the 106 face landmarks on the face image. Note, you should not run any inference functions on the image after calling this method.
- Parameters
- Parameters
tf_image – the input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.landmarks – the 106 face landmarks.
color – the color to use for the annotation (default = ColorRGB(0, 255, 255).
- Scale
size of the landmark points draw on the face. Increase scale factor for higher resolution images.
- Returns
Error code, see
ERRORCODE
.
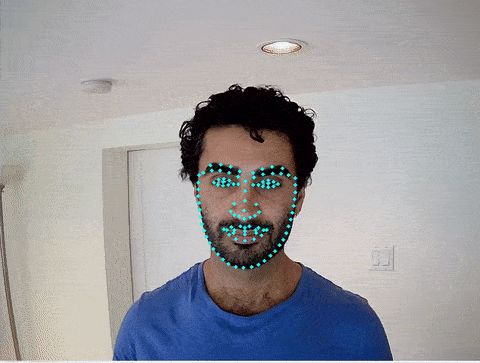
- SDK.draw_head_orientation_axes(self: tfsdk.SDK, tf_image: tfsdk.TFImage, face_box_and_landmarks: tfsdk.FaceBoxAndLandmarks, yaw: float, pitch: float, roll: float, scale: int = 2) → tfsdk.ERRORCODE¶
Draw the 3D face orientation axes. Note, you should not run any inference functions on the image after calling this method.
- Parameters
- Parameters
tf_image – the input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.face_box_and_landmarks – the face for which the orientation axes will be drawn.
yaw – the yaw in radians.
pitch – the pitch in radians.
roll – the roll in radians.
- Scale
sale factor for the axis line thickness and length. Increase scale factor for higher resolution images.
- Returns
Error code, see
ERRORCODE
.
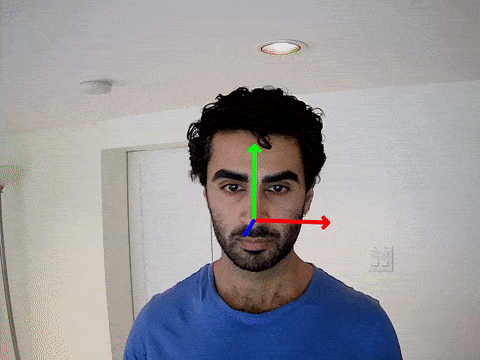
- SDK.draw_head_orientation_box(self: tfsdk.SDK, tf_image: tfsdk.TFImage, rotation_vec: Annotated[List[float], FixedSize(3)], translation_vec: Annotated[List[float], FixedSize(3)], scale: int = 2) → tfsdk.ERRORCODE¶
Draw 3D box on the face. Note, you should not run any inference functions on the image after calling this method.
- Parameters
- Parameters
tf_image – the input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.rotation_vec – the rotation vector, returned by
tfsdk.estimate_head_orientation()
.translation_vec – the translation vector, returned by
tfsdk.estimate_head_orientation()
.
- Scale
sale factor for the box line thickness. Increase scale factor for higher resolution images.
- Returns
Error code, see
ERRORCODE
.
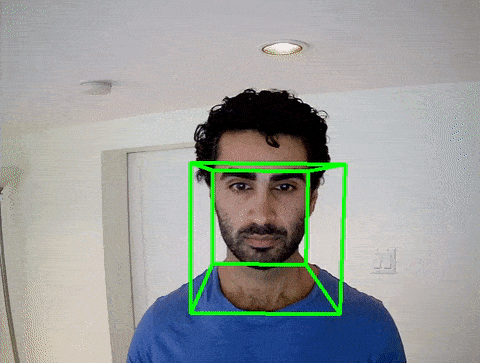
- SDK.draw_candidate_bounding_box_and_label(self: tfsdk.SDK, tf_image: tfsdk.TFImage, face_box_and_landmarks: tfsdk.FaceBoxAndLandmarks, candidate: tfsdk.Candidate, color: tfsdk.ColorRGB = <tfsdk.ColorRGB object at 0x7f037538e230>, scale: int = 2) → tfsdk.ERRORCODE¶
Draw a bounding box around the face and a label containing the identity and match probability. Note, you should not run any inference functions on the image after calling this method.
- Parameters
- Parameters
tf_image – the input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.face_box_and_landmarks – the face to annotate.
candidate – the candidate information.
color – the color to use for the annotation (default = ColorRGB(255, 255, 255).
- Scale
scale factor for the bounding box thickness. Increase scale factor for higher resolution images.
- Returns
Error code, see
ERRORCODE
.
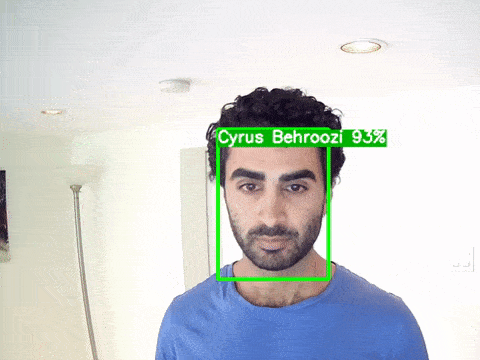
- SDK.draw_object_labels(self: tfsdk.SDK, tf_image: tfsdk.TFImage, bounding_boxes: List[tfsdk.BoundingBox], scale: int = 2) → tfsdk.ERRORCODE¶
Draw object detector bounding boxes and labels on the image. Note, you should not run any inference functions on the image after calling this method.
- Parameters
- Parameters
tf_image – the input
tfsdk.TFImage
, returned bytfsdk.SDK.preprocess_image()
.bounding_boxes – the bounding boxes returned by
tfsdk.SDK.detect_objects()
.scale – scale factor for the text size and bounding box thickness. Increase scale factor for higher resolution images.
- Returns
Error code, see
ERRORCODE
.
- SDK.draw_pose(self: tfsdk.SDK, tf_image: tfsdk.TFImage, landmarks: List[List[tfsdk.Landmark]]) → tfsdk.ERRORCODE¶
- Draw body pose on the image.
Note, you should not run any inference functions on the image after calling this method.
- Parameters
tf_image – the input
tfsdk.TFImage
on which to draw the pose.landmarks – a list of
tfsdk.Landmark
returned bytfsdk.SDK.estimate_pose()
.
- ColorRGB.__init__(*args, **kwargs)¶
Overloaded function.
__init__(self: tfsdk.ColorRGB) -> None
__init__(self: tfsdk.ColorRGB, r: int, g: int, b: int) -> None