Image Annotation
Methods for annotating images with face landmarks, bounding boxes, face recognition labels, and more. After annotating an image, you must not run inference functions on that image. You can call multiple annotation methods on the same image.
-
ErrorCode Trueface::SDK::drawFaceBoxAndLandmarks(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, bool includeLandmarks = false, const ColorRGB &color = ColorRGB(0, 255, 255), unsigned int scale = 2)
Draw face detection bounding box and 5 keypoint landmarks. Note, you should not run any inference functions on the image after calling this method.
- Parameters:
tfImage – [in] The input image on which to draw the labels.
faceBoxAndLandmarks – [in] The face to annotate.
includeLandmarks – [in] Indicates if the 5 keypoint landmarks should also be drawn.
color – [in] The color to use for the annotation.
scale – [in] Scale factor for the bounding box thickness. Increase scale factor for higher resolution images.
- Returns:
Error code, see ErrorCode.
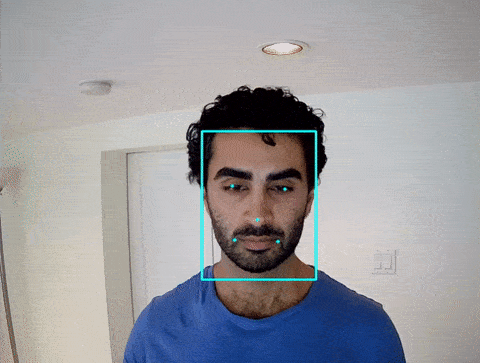
-
ErrorCode Trueface::SDK::drawFaceLandmarks(const TFImage &tfImage, const Landmarks &landmarks, const ColorRGB &color = ColorRGB(0, 255, 255), unsigned int scale = 2)
Draw the 106 face landmarks on the face image. Note, you should not run any inference functions on the image after calling this method.
- Parameters:
tfImage – [in] The input image on which to draw the landmarks.
landmarks – [in] The 106 face landmarks.
color – [in] The color to use for the annotation.
scale – [in] Size of the landmark points draw on the face. Increase scale factor for higher resolution images.
- Returns:
error code
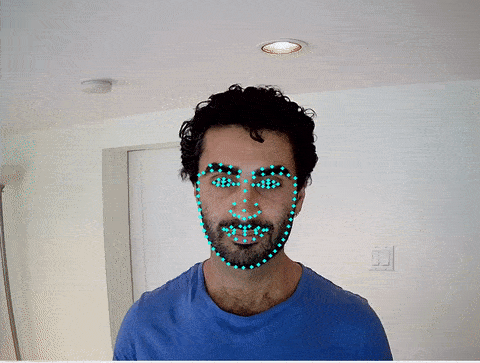
-
ErrorCode Trueface::SDK::drawHeadOrientationAxes(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, const HeadOrientation &headOrientation, unsigned int scale = 2)
Draw the 3D face orientation axes. Note, you should not run any inference functions on the image after calling this method.
- Parameters:
tfImage – [in] The input image on which to draw the rotation axes.
faceBoxAndLandmarks – [in] The face for which the orientation axes will be drawn.
headOrientation – [in] The head orientation, returned by estimateHeadOrientation().
scale – [in] The scale factor for the axis line thickness and length. Increase scale factor for higher resolution images.
- Returns:
Error code.
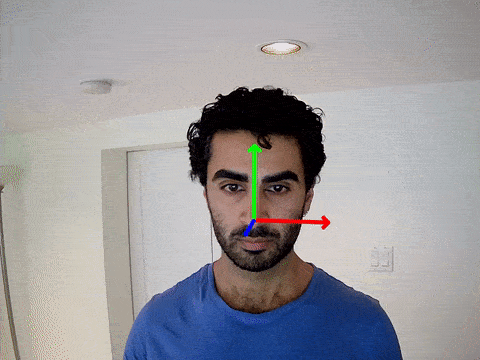
-
ErrorCode Trueface::SDK::drawHeadOrientationBox(const TFImage &tfImage, const HeadOrientation &headOrientation, unsigned int scale = 2)
Draw 3D box on the face. Note, you should not run any inference functions on the image after calling this method.
- Parameters:
tfImage – [in] The input image on which to draw the rotation axes.
headOrientation – [in] The head orientation, returned by estimateHeadOrientation().
scale – [in] The scale factor for the box line thickness. Increase scale factor for higher resolution images.
- Returns:
Error code.
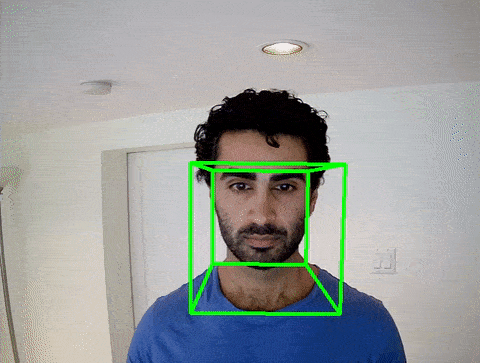
-
ErrorCode Trueface::SDK::drawCandidateBoundingBoxAndLabel(const TFImage &tfImage, const FaceBoxAndLandmarks &faceBoxAndLandmarks, const Candidate &candidate, const ColorRGB &color = ColorRGB(0, 255, 0), unsigned int scale = 2)
Draw a bounding box around the face and a label containing the identity and match probability. Note, you should not run any inference functions on the image after calling this method.
- Parameters:
tfImage – [in] The input image on which to draw the labels.
faceBoxAndLandmarks – [in] The face to annotate.
candidate – [in] The candidate information.
color – [in] The color to use for the annotation.
scale – [in] The scale factor for the text size and bounding box thickness. Increase scale factor for higher resolution images.
- Returns:
ErrorCode.
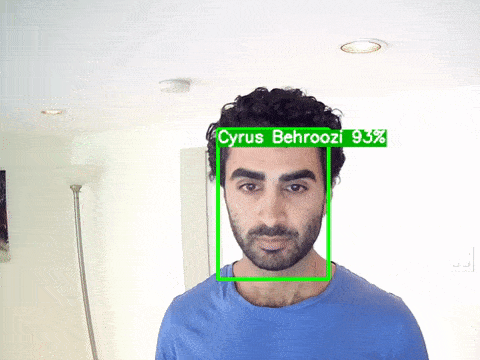
-
ErrorCode Trueface::SDK::drawObjectLabels(const TFImage &tfImage, const std::vector<BoundingBox> &boundingBoxes, unsigned int scale = 2)
Draw object detector bounding boxes and labels on the image. Note, you should not run any inference functions on the image after calling this method.
- Parameters:
tfImage – [in] The input image on which to draw the labels.
boundingBoxes – [in] The bounding boxes returned by detectObjects().
scale – [in] Scale factor for the text size and bounding box thickness. Increase scale factor for higher resolution images.
- Returns:
Error code, see ErrorCode.